
项目设计大概思路:
1.游戏的菜单界面的编写(Play、Score、About) 添加背景音乐->选项场景的创建->设置关闭声音->游戏测试
2.游戏舞台屏幕的编写,背景无限移动
3.飞船类的编写
4.敌机的编写
5.子弹类以及碰撞检测的编写
6.游戏胜利以及失败场景的编写
详细设计:
1.主场景(菜单选项场景)的设计
HMenu.h:
[plain]
- //
- // HMenu.h
- // dafeiji
- //
- // Created by 丁小未 on 13-9-25.
- //
- //
- #ifndef __dafeiji__HMenu__
- #define __dafeiji__HMenu__
- #include <iostream>
- #include "cocos2d.h"
- using namespace cocos2d;
- class HMenu : public CCLayer
- {
- public:
- virtual bool init();
- static CCScene * scene();
- CREATE_FUNC(HMenu);
- private:
- //开始游戏
- void playerIsPressed();
- //分数显示
- void scoreIsPressed();
- //关于作者
- void aboutIsPressed();
- };
- #endif /* defined(__dafeiji__HMenu__) */
HMenu.cpp:
[plain]
- //
- // HMenu.cpp
- // dafeiji
- //
- // Created by 丁小未 on 13-9-25.
- //
- //
- #include "HMenu.h"
- #include "SimpleAudioEngine.h"
- #include "HScore.h"
- #include "HAbout.h"
- #include "HWorld.h"
- using namespace cocos2d;
- using namespace CocosDenshion;
- CCScene * HMenu::scene()
- {
- CCScene *scene = CCScene::create();
- HMenu * layer = HMenu::create();
- scene->addChild(layer);
- return scene;
- }
- bool HMenu::init()
- {
- if (!CCLayer::init()) {
- return false;
- }
- SimpleAudioEngine::sharedEngine()->playBackgroundMusic("menuMusic", true);
- CCSize size = CCDirector::sharedDirector()->getWinSize();
- //添加背景精灵
- CCSprite *sp = CCSprite::create("menu_bg.png");
- sp->setPosition(CCPointMake(size.width/2, size.height/2));
- addChild(sp);
- //添加三个菜单选项贴图
- CCMenuItemImage *itemPlay = CCMenuItemImage::create("play_nor.png", "play_pre.png",this,menu_selector(HMenu::playerIsPressed));
- CCMenuItemImage * itemScore = CCMenuItemImage::create("score_nor.png", "score_pre.png", this, menu_selector(HMenu::scoreIsPressed));
- itemScore->setPosition(CCPointMake(0, -itemScore->getContentSize().height - 20));
- CCMenuItemImage * itemAbout = CCMenuItemImage::create("about_nor.png", "about_pre.png",this,menu_selector(HMenu::aboutIsPressed));
- itemAbout->setPosition(CCPointMake(0, -itemAbout->getContentSize().height*2 - 40));
- //添加菜单选项卡
- CCMenu *menu = CCMenu::create(itemPlay,itemScore,itemAbout,NULL);
- addChild(menu);
- return true;
- }
- //进入玩游戏主场景
- void HMenu::playerIsPressed()
- {
- CCLog("1");
- CCDirector::sharedDirector()->replaceScene(CCTransitionFadeDown::create(1, HWorld::scene()));
- }
- //进入显示分数场景
- void HMenu::scoreIsPressed()
- {
- CCLog("2");
- CCDirector::sharedDirector()->replaceScene(CCTransitionCrossFade::create(1, HScore::scene()));
- }
- //进入关于作者场景
- void HMenu::aboutIsPressed()
- {
- CCLog("3");
- CCDirector::sharedDirector()->replaceScene(CCTransitionCrossFade::create(1, HAbout::scene()));
- }
2.游戏背景的设计(实现无限滚屏,也就是两张图片来回不停切换)
HMap.h:
[plain]
- //
- // HMap.h
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #ifndef __dafeiji__HMap__
- #define __dafeiji__HMap__
- #include <iostream>
- #include "cocos2d.h"
- using namespace cocos2d;
- typedef enum
- {
- tag_oneImg,
- tag_twoImg,
- }tagMap;
- class HMap:public CCLayer
- {
- public:
- //创建一个map
- static HMap* createMap(const char * fileName);
- private:
- void mapInit(const char * fileName);
- void update(float time);
- virtual void onExit();
- };
- #endif /* defined(__dafeiji__HMap__) */
HMap.cpp:
[plain]
- //
- // HMap.cpp
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #include "HMap.h"
- //模仿CREATE_FUNC()函数自己创建一个对象
- HMap * HMap::createMap(const char *fileName)
- {
- HMap * map = new HMap();
- if (map&&map->create()) {
- map->autorelease();
- map->mapInit(fileName);
- return map;
- }
- CC_SAFE_DELETE(map);
- return NULL;
- }
- void HMap::mapInit(const char *fileName)
- {
- CCSize size = CCDirector::sharedDirector()->getWinSize();
- //创建两张图片精灵来回切换
- CCSprite * turnImg1 = CCSprite::create(fileName);
- turnImg1->setPosition(CCPointMake(turnImg1->getContentSize().width/2, turnImg1->getContentSize().height/2));
- this->addChild(turnImg1,0,tag_oneImg);
- CCSprite * turnImg2 = CCSprite::create(fileName);
- turnImg2->setPosition(CCPointMake(turnImg2->getContentSize().width/2, turnImg2->getContentSize().height * 1.5));
- this->addChild(turnImg2,0,tag_twoImg);
- //启动定时器来实现两张背景图片的循环交叉滚动
- this->scheduleUpdate();
- }
- //自上而下滚屏效果
- void HMap::update(float time)
- {
- CCSize size = CCDirector::sharedDirector()->getWinSize();
- CCSprite * sp1 = (CCSprite *)this->getChildByTag(tag_oneImg);
- //如果第一张背景图的中点到达屏幕下方背景图高度的一半的时候(也就是第一张图片移除图片下面的时候)重新设置他的位置到屏幕上面,图片下边缘跟手机屏幕上边缘重合-1个像素
- if (sp1->getPositionY()<=-sp1->getContentSize().height/2) {
- sp1->setPosition(CCPointMake(sp1->getContentSize().width/2, sp1->getContentSize().height*1.5f - 1));
- }
- //如果还没需要换位置就让他向下移动一个像素
- else
- {
- sp1->setPosition(ccpAdd(sp1->getPosition(), ccp(0,-1)));
- }
- CCSprite * sp2 = (CCSprite *)this->getChildByTag(tag_twoImg);
- //如果第二张背景图移出屏幕最下方则重新设置他的位置在屏幕的最上方
- if (sp2->getPositionY()<=-sp2->getContentSize().height/2) {
- sp2->setPosition(CCPointMake(sp2->getContentSize().width/2, sp2->getContentSize().height*1.5 - 1));
- }
- //向下移动
- else{
- sp2->setPosition(ccpAdd(sp2->getPosition(), ccp(0,-1)));
- }
- }
- //如果退出
- void HMap::onExit()
- {
- this->unscheduleUpdate();
- CCLayer::onExit();//还要调用父类的退出方法
- }
3.主角类的设计:
HPlayer.h:
[plain]
- //
- // HPlayer.h
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #ifndef __dafeiji__HPlayer__
- #define __dafeiji__HPlayer__
- #include <iostream>
- #include "cocos2d.h"
- #include <sstream>
- using namespace std; //导入C++命名空间
- using namespace cocos2d;
- //模板,将任意类型转化成string类型
- template <typename T>
- string Convert2String(const T &value)
- {
- stringstream ss;
- ss<<value;
- return ss.str();
- }
- //创建player,具备点击的精灵
- class HPlayer: public CCSprite,public CCTouchDelegate
- {
- public:
- //创建主角精灵
- static HPlayer * createPlayer(const char* fileName);
- //当前血量
- int hp;
- //最大血量
- int hpMax;
- //分数
- int score;
- //杀敌数
- int killCount;
- //掉血
- void downHp();
- //添加分数
- void addScore(float _value);
- //添加杀敌数
- void addKillCount(float _value);
- //判断是否死亡
- bool isDead;
- //private:
- //无敌状态的时间
- int strongTime;
- //是否无敌状态
- bool isStrong;
- //
- int strongCount;
- //无敌状态函数
- void strongIng();
- //主角精灵初始化
- void playerInit();
- virtual void onEnter();
- virtual void onExit();
- virtual bool ccTouchBegan(CCTouch *pTouch, CCEvent *pEvent);
- virtual void ccTouchMoved(CCTouch *pTouch, CCEvent *pEvent);
- virtual void ccTouchEnded(CCTouch *pTouch, CCEvent *pEvent);
- };
- #endif /* defined(__dafeiji__HPlayer__) */
HPlayer.cpp:
[plain]
- //
- // HPlayer.cpp
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #include "HPlayer.h"
- #include "HWorld.h"
- using namespace cocos2d;
- //创建主角精灵
- HPlayer *HPlayer::createPlayer(const char* fileName)
- {
- HPlayer * player=new HPlayer();
- //由于继承自CCSprite,然后调用CCSprite的initWithFile方法
- if (player && player->initWithFile(fileName)) {
- player->autorelease();
- //调用初始化方法
- player->playerInit();
- return player;
- }
- CC_SAFE_DELETE(player);
- return NULL;
- }
- //设置配置参数,初始化血量,杀敌数等
- void HPlayer::playerInit()
- {
- CCSize size=CCDirector::sharedDirector()->getWinSize();
- //设置主角的位置在屏幕下面正中央
- this->setPosition(ccp(size.width*0.5, this->getContentSize().height*0.5));
- //设置参数(血量,当前血量,无敌时间,分数)
- hpMax=5;
- hp=5;
- score=0;
- strongTime=2*60;
- //添加右下角的血量贴图
- for (int i=0; i<5; i++) {
- CCSprite *spHp=CCSprite::create("icon_hp.png");
- //设置每个血量贴图在屏幕的右下角一次排列,并且从右至左tag分别是值为5,4,3,2,1的枚举类型
- spHp->setPosition(ccp(size.width-this->getContentSize().width*0.5*i-20, spHp->getContentSize().height*0.5));
- if (i==0) {
- spHp->setTag(tag_playerHP1);
- }else if(i==1){
- spHp->setTag(tag_playerHP2);
- }else if(i==2){
- spHp->setTag(tag_playerHP3);
- }else if(i==3){
- spHp->setTag(tag_playerHP4);
- }else if(i==4){
- spHp->setTag(tag_playerHP5);
- }
- //将每一个血量贴图添加到HWorld场景中
- HWorld::sharedWorld()->addChild(spHp,10);
- }
- //添加分数label
- CCLabelTTF *label=CCLabelTTF::create("分数", "Helvetica-Blood", 24);
- label->setPosition(ccp(30, size.height-22)); //位置左上方
- HWorld::sharedWorld()->addChild(label,10);
- //分数结果
- string strScore=Convert2String(score);
- CCLabelTTF * labelScores=CCLabelTTF::create(strScore.c_str(),"Helvetica-Blood",24);
- labelScores->setPosition(ccp(110, size.height-22));
- labelScores->setColor(ccc3(255, 255, 0)); //设置颜色为黄色
- HWorld::sharedWorld()->addChild(labelScores,10,tag_scoreTTF);
- //添加杀敌label
- CCLabelTTF *labelKill=CCLabelTTF::create("杀敌", "Helvetica-Blood", 24);
- labelKill->setPosition(ccp(30, size.height-52));
- HWorld::sharedWorld()->addChild(labelKill,10);
- //杀敌数
- string strKillCount=Convert2String(killCount);
- strKillCount+="/100"; //C++中string可以直接拼接
- CCLabelTTF *labelKillCount=CCLabelTTF::create(strKillCount.c_str(), "Helvetica-Blood", 24);
- labelKillCount->setPosition(ccp(110, size.height-52));
- labelKillCount->setColor(ccc3(255, 255, 0)); //设置为黄色
- HWorld::sharedWorld()->addChild(labelKillCount,10,tag_killsCountTTF);
- }
- //添加分数
- void HPlayer::addScore(float _value)
- {
- score+=_value;
- string strScore=Convert2String(score);
- //根据HWorld获取场景中分数标签并且改变他的值
- CCLabelTTF *ttf=(CCLabelTTF *)HWorld::sharedWorld()->getChildByTag(tag_scoreTTF);
- ttf->setString(strScore.c_str());
- }
- //添加杀敌数
- void HPlayer::addKillCount(float _value)
- {
- killCount+=_value;
- string strKillCount=Convert2String(killCount);
- strKillCount+="/100";
- //获取HWorld中杀敌数标签,并且改变他的值
- CCLabelTTF *ttf=(CCLabelTTF *)HWorld::sharedWorld()->getChildByTag(tag_killsCountTTF);
- ttf->setString(strKillCount.c_str());
- //当杀敌过百的时候,游戏胜利
- if (killCount>=100) {
- //
- int oldScore=atoi(CCUserDefault::sharedUserDefault()->getStringForKey("user_score","-1").c_str());//当取出的键值为NULL的时候默认为1
- if (oldScore!=-1&&score>oldScore) {
- CCUserDefault::sharedUserDefault()->setStringForKey("user_score", Convert2String(score));
- CCUserDefault::sharedUserDefault()->flush(); //必须要写,刷新数据
- }
- //调用胜利界面
- HWorld::sharedWorld()->winGame();
- }
- }
- //让主角掉血的方法,分为死亡和飞死亡两种情况来分析
- void HPlayer::downHp()
- {
- //如果还出于无敌状态则不需要掉血
- if (isStrong) {
- return;
- }
- //血量减少1
- hp-=1;
- //如果血量少于0,则将主角设置不可见并且设置他已经死亡
- if (hp<=0) {
- this->setVisible(false);
- isDead=true;
- //取出旧的分数
- int oldScore=atoi(CCUserDefault::sharedUserDefault()->getStringForKey("user_score","-1").c_str());
- //当有新的分数,就将新的分数保存到数据中,并且要刷新保存
- if (oldScore!=-1&&score>oldScore)
- {
- CCUserDefault::sharedUserDefault()->setStringForKey("user_score", Convert2String(score));
- CCUserDefault::sharedUserDefault()->flush();
- }
- HWorld::sharedWorld()->lostGame();
- }
- //如果主角还为死亡然后移除相应界面中的血量贴图
- else
- {
- switch (hp) {
- case 1:
- HWorld::sharedWorld()->removeChildByTag(tag_playerHP2, true);
- break;
- case 2:
- HWorld::sharedWorld()->removeChildByTag(tag_playerHP3, true);
- break;
- case 3:
- HWorld::sharedWorld()->removeChildByTag(tag_playerHP4, true);
- break;
- case 4:
- HWorld::sharedWorld()->removeChildByTag(tag_playerHP5, true);
- break;
- }
- //将无敌状态开启,并且开启无敌状态的定时器
- isStrong=true;
- strongCount=0;//无敌状态的时间
- this->schedule(schedule_selector(HPlayer::strongIng));
- }
- }
- //正处于无敌状态中
- void HPlayer::strongIng()
- {
- strongCount++;
- //当无敌状态时间到的时候,取消无敌状态,并设置为可见,并且取消定时器
- if (strongCount%strongTime==0) {
- this->setVisible(true);
- isStrong=false;
- this->unschedule(schedule_selector(HPlayer::strongIng));
- }
- else //如果是无敌状态,让他不断的闪烁
- {
- if (strongCount%3==0) {
- this->setVisible(false);
- }else{
- this->setVisible(true);
- }
- }
- }
- //当主角精灵初始化的时候注册他的触摸事件
- void HPlayer::onEnter()
- {
- CCDirector::sharedDirector()->getTouchDispatcher()->addTargetedDelegate(this, 0, true);
- CCSprite::onEnter(); //调用父类的onEnter方法,这里是调用的CCNode的onEnter方法
- }
- //当主角精灵退出的时候取消他的注册触摸事件
- void HPlayer::onExit()
- {
- CCDirector::sharedDirector()->getTouchDispatcher()->removeDelegate(this);
- CCSprite::onExit();
- }
- //设置主角精灵的当前位置
- bool HPlayer::ccTouchBegan(CCTouch *pTouch, CCEvent *pEvent)
- {
- this->setPosition(pTouch->getLocation());
- return true;
- }
- //触摸移动的时候不断的去移动主角精灵让他跟随着鼠标移动而移动
- void HPlayer::ccTouchMoved(CCTouch *pTouch, CCEvent *pEvent)
- {
- this->setPosition(pTouch->getLocation());
- }
- //触摸结束
- void HPlayer::ccTouchEnded(CCTouch *pTouch, CCEvent *pEvent)
- {
- this->setPosition(pTouch->getLocation());
- }
4.敌人类的设计:
HEnemy.h:
[plain]
- //
- // HEenemy.h
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #ifndef __dafeiji__HEenemy__
- #define __dafeiji__HEenemy__
- #include <iostream>
- #include "cocos2d.h"
- using namespace cocos2d;
- class HEnemy:public CCSprite
- {
- public:
- //创建Enemy
- static HEnemy* createEnemy(const char *fileName,int _type);
- //怪物的值
- int scoreValue;
- private:
- void enemyInit(const char * fileName,int _type);
- void createAnimate(const char *fileName,int allCount);
- void update(float time);
- //是否被攻击了
- bool isActed;
- int type;
- };
- #endif /* defined(__dafeiji__HEenemy__) */
HEnemy.cpp:
[plain]
- //
- // HEenemy.cpp
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #include "HEenemy.h"
- #include "HWorld.h"
- using namespace cocos2d;
- //创建Enemy
- HEnemy* HEnemy::createEnemy(const char *fileName, int _type)
- {
- HEnemy * enemy = new HEnemy();
- if (enemy && enemy->initWithFile(fileName)) {
- enemy->autorelease();
- enemy->enemyInit(fileName, _type);
- return enemy;
- }
- CC_SAFE_DELETE(enemy);
- return NULL;
- }
- void HEnemy::enemyInit(const char *fileName, int _type)
- {
- type = _type;
- createAnimate(fileName, 10);//创建动画
- CCSize size = CCDirector::sharedDirector()->getWinSize();
- if (_type == 0) {
- scoreValue = 198;
- }
- else if(_type == 1)
- {
- scoreValue = 428;
- }
- else if(_type == 2)
- {
- scoreValue = 908;
- }
- this->setPosition(CCPointMake(CCRANDOM_0_1()*size.width, size.height+this->getContentSize().height));
- this->scheduleUpdate();
- }
- void HEnemy::update(float time)
- {
- switch (type)
- {
- case 0:
- {
- this->setPosition(ccpAdd(this->getPosition(), ccp(0,-3)));
- break;
- }
- case 1:
- {
- if (isActed) {
- break;
- }
- isActed = true;
- this->runAction(CCSequence::create(CCMoveTo::create(1.6, HWorld::sharedWorld()->getPlayer()->getPosition()),CCDelayTime::create(1.0),CCMoveTo::create(1.8, this->getPosition()),NULL));
- break;
- }
- }
- if (this->getPositionY()<-this->getContentSize().height) {
- HWorld::sharedWorld()->getArrayForEnemy()->removeObject(this);
- this->getParent()->removeChild(this,true);
- }
- HPlayer * player = HWorld::sharedWorld()->getPlayer();
- if (!player->isDead) {
- if (player->boundingBox().intersectsRect(this->boundingBox())) {
- player->downHp();
- }
- }
- }
- //创建动画
- void HEnemy::createAnimate(const char *fileName, int allCount)
- {
- CCAnimation *animation = CCAnimation::create();
- CCTexture2D *texture = CCTextureCache::sharedTextureCache()->addImage(fileName);
- int eachWith = this->getContentSize().width/allCount;
- for (int i = 0; i<allCount; i++) {
- animation->addSpriteFrameWithTexture(texture, CCRectMake(i*eachWith, 0, eachWith, this->getContentSize().height));
- }
- animation->setDelayPerUnit(0.03f);
- animation->setRestoreOriginalFrame(true);
- animation->setLoops(-1);
- CCFiniteTimeAction *animate = CCAnimate::create(animation);
- this->runAction(animate);
- }
5.子弹类的设计:
HBullet.h:
[plain]
- //
- // HBullet.h
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #ifndef __dafeiji__HBullet__
- #define __dafeiji__HBullet__
- #include <iostream>
- #include "cocos2d.h"
- using namespace cocos2d;
- class HBullet:public CCSprite
- {
- public:
- //创建子弹
- static HBullet * createBullet(const char *_fileName,float _speed,CCPoint _position);
- private:
- //子弹的初始化,供create调用
- void bulletInit(float _speed,CCPoint _position);
- void update(float time);
- float speed;
- virtual void onExit();
- };
- #endif /* defined(__dafeiji__HBullet__) */
HBullet.cpp:
[plain]
- //
- // HBullet.cpp
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #include "HBullet.h"
- #include "HWorld.h"
- #include "HEenemy.h"
- #include "SimpleAudioEngine.h"
- using namespace CocosDenshion;
- HBullet *HBullet::createBullet(const char *_fileName,float _speed, CCPoint _position)
- {
- HBullet *bullet=new HBullet();
- if (bullet && bullet->initWithFile(_fileName)) {
- bullet->autorelease();
- bullet->bulletInit(_speed, _position);
- return bullet;
- }
- CC_SAFE_DELETE(bullet);
- return NULL;
- }
- //初始化子弹的速度以及位置,并且调用子弹的update方法
- void HBullet::bulletInit(float _speed,CCPoint _position)
- {
- speed=_speed;
- this->setPosition(_position);
- this->scheduleUpdate();
- }
- //碰撞检测
- void HBullet::update(float time)
- {
- //不断的改变子弹的位置(当前坐标+子弹的速度)
- this->setPosition(ccpAdd(this->getPosition(),ccp(0, speed)));
- //获取敌人对象数组
- CCArray *array=HWorld::sharedWorld()->getArrayForEnemy();
- //依次的遍历敌人进行碰撞检测
- for (int i=0; i<array->count(); i++)
- {
- //获取一个敌人对象
- HEnemy *enemy=(HEnemy *)array->objectAtIndex(i);
- //如果两个有碰撞
- if (enemy->boundingBox().intersectsRect(this->boundingBox()))
- {
- //调用击中音效
- SimpleAudioEngine::sharedEngine()->playEffect("effect_boom.mp3");
- //播放击中的爆破效果
- CCParticleSystemQuad *particle=CCParticleSystemQuad::create("particle_boom.plist");
- particle->setPosition(enemy->getPosition());
- //将爆破效果执行结束后移除
- particle->setAutoRemoveOnFinish(true);
- //将爆破效果添加到主场景中
- HWorld::sharedWorld()->addChild(particle);
- //调用主场景中获取主角的方法并调用他的addScore方法
- HWorld::sharedWorld()->getPlayer()->addScore(enemy->scoreValue);
- HWorld::sharedWorld()->getPlayer()->addKillCount(1);
- //从数组中移除到当前的敌人
- array->removeObject(enemy);
- //从界面中移除敌人
- HWorld::sharedWorld()->removeChild(enemy, true);
- //移除当前的子弹类
- HWorld::sharedWorld()->removeChild(this, true);
- }
- }
- }
- //退出的时候将子弹的Update方法取消
- void HBullet::onExit()
- {
- this->unscheduleUpdate();
- //注意要调用父类的退出方法
- CCSprite::onExit();
- }
6.游戏Fighting主场景的设计
HWorld.h:
[plain]
- //
- // HWorld.h
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #ifndef __dafeiji__HWorld__
- #define __dafeiji__HWorld__
- #include <iostream>
- #include "cocos2d.h"
- #include "HPlayer.h"
- typedef enum
- {
- //主角的tag
- tag_player,
- //5个血量的tag
- tag_playerHP1,
- tag_playerHP2,
- tag_playerHP3,
- tag_playerHP4,
- tag_playerHP5,
- //分数的Label的tag
- tag_scoreTTF,
- //杀敌数的tag
- tag_killsCountTTF,
- }tagWorld;
- using namespace cocos2d;
- class HWorld : public CCLayer{
- public:
- static CCScene * scene();
- static HWorld * sharedWorld();
- //获取玩家精灵
- HPlayer *getPlayer();
- //获取敌人数组
- CCArray *getArrayForEnemy();
- void lostGame();
- void winGame();
- private:
- virtual bool init();
- CREATE_FUNC(HWorld);
- virtual ~HWorld();
- HWorld();
- void backMenu();//返回菜单
- CCArray *arrayEnemy;
- void autoCreateEnemy();
- void autoCreateBullet();
- };
- #endif /* defined(__dafeiji__HWorld__) */
HWorld.cpp:
[plain]
- //
- // HWorld.cpp
- // dafeiji
- //
- // Created by 丁小未 on 13-9-26.
- //
- //
- #include "HWorld.h"
- #include "SimpleAudioEngine.h"
- #include "HMap.h"
- #include "HPlayer.h"
- #include "HMenu.h"
- #include "HBullet.h"
- #include "HEenemy.h"
- using namespace CocosDenshion;
- static HWorld *sh ;
- HWorld* HWorld::sharedWorld()
- {
- if (sh!= NULL) {
- return sh;
- }
- return NULL;
- }
- CCScene * HWorld::scene()
- {
- CCScene * scene = CCScene::create();
- HWorld * layer = HWorld::create();
- scene->addChild(layer);
- return scene;
- }
- bool HWorld::init()
- {
- if (!CCLayer::init()) {
- return false;
- }
- //获取到当前的对象
- sh = this;
- //播放场景音乐
- SimpleAudioEngine::sharedEngine()->playBackgroundMusic("gameMusic.mp3", true);
- //添加背景
- HMap * map = HMap::createMap("map.png");
- addChild(map);
- //添加主角精灵
- HPlayer * player = HPlayer::createPlayer("player.png");
- addChild(player,0,tag_player);
- //创建子弹
- this->schedule(schedule_selector(HWorld::autoCreateBullet),0.3);
- //创建敌人
- this->schedule(schedule_selector(HWorld::autoCreateEnemy),1);
- //创建arrayEnemy
- arrayEnemy = CCArray::create();
- CC_SAFE_RETAIN(arrayEnemy);
- return true;
- }
- //自动创建不同的敌人
- void HWorld::autoCreateEnemy()
- {
- int randomCount=CCRANDOM_0_1()*10;
- for (int i=0; i<randomCount; i++)
- {
- int random=CCRANDOM_0_1()*10;
- HEnemy *enemy=NULL;
- int randomType=CCRANDOM_0_1()*10;
- const char *name;
- if (random>=0&&random<=2)
- {
- name="enemy_bug.png";
- }else if(random>=3&&random<=6)
- {
- name="enemy_duck.png";
- }else if(random>=7&&random<=10)
- {
- name="enemy_pig.png";
- }
- if (randomType%2==0) {
- randomType=0;
- }else
- {
- randomType=1;
- }
- enemy=HEnemy::createEnemy(name, randomType);
- arrayEnemy->addObject(enemy);
- addChild(enemy);
- }
- }
- //自动创建子弹
- void HWorld::autoCreateBullet()
- {
- HPlayer *player=(HPlayer *)this->getChildByTag(tag_player);
- this->addChild(HBullet::createBullet("p_bullet.png", 1, ccpAdd(player->getPosition(), ccp(0, player->getContentSize().height*0.5))));
- SimpleAudioEngine::sharedEngine()->playEffect("effect_bullet.mp3");
- }
- //游戏胜利场景
- void HWorld::winGame()
- {
- CCSize size = CCDirector::sharedDirector()->getWinSize();
- //创建一个带有颜色的层
- CCLayerColor *layer = CCLayerColor::create(ccc4(0, 0, 0, 190),size.width,size.height);
- //创建背景图片
- CCSprite * sp = CCSprite::create("game_win.png");
- sp->setPosition(CCPointMake(size.width/2, size.height/2));
- layer->addChild(sp);
- addChild(layer,100);
- //添加菜单项
- CCLabelTTF * ttback = CCLabelTTF::create("返回主菜单", "Helvetica-Bold", 23);
- CCMenuItemLabel *menuLabel = CCMenuItemLabel::create(ttback, this, menu_selector(HWorld::backMenu));
- menuLabel->setPosition(CCPointMake(0, -200));
- CCMenu * menu = CCMenu::create(menuLabel,NULL);
- addChild(menu,100);
- //游戏暂停
- CCDirector::sharedDirector()->pause();
- }
- //游戏失败场景
- void HWorld::lostGame()
- {
- CCSize size = CCDirector::sharedDirector()->getWinSize();
- //创建有色层
- CCLayerColor * layer = CCLayerColor::create(ccc4(0, 0, 0, 190), size.width, size.height);
- CCSprite *sp = CCSprite::create("game_lost.png");
- sp->setPosition(CCPointMake(size.width/2, size.height/2));
- //将图片添加到层中
- layer->addChild(sp);
- //将层添加到场景中
- this->addChild(layer,100);
- CCLabelTTF *ttback = CCLabelTTF::create("返回主菜单", "Helvetica-Bold", 23);
- CCMenuItemLabel *menuLabel = CCMenuItemLabel::create(ttback, this, menu_selector(HWorld::backMenu));
- //菜单选项卡在CCMenu中的坐标系是以屏幕的中点为原点
- menuLabel->setPosition(CCPointMake(0, -200));
- CCMenu* menu = CCMenu::create(menuLabel,NULL);
- addChild(menu,100);
- CCDirector::sharedDirector()->pause();
- }
- //通过tag获取主角精灵
- HPlayer * HWorld::getPlayer()
- {
- HPlayer * player=(HPlayer *)HWorld::sharedWorld()->getChildByTag(tag_player);
- return player;
- }
- //返回敌人数组
- CCArray * HWorld::getArrayForEnemy()
- {
- return arrayEnemy;
- }
- //返回菜单
- void HWorld::backMenu()
- {
- this->unscheduleAllSelectors();
- CCDirector::sharedDirector()->resume();
- CCDirector::sharedDirector()->replaceScene(HMenu::scene());
- }
- //构造函数
- HWorld::HWorld(){}
- //析构函数
- HWorld::~HWorld()
- {
- //清除敌人数组
- }
7.分数场景的设计:
HScore.h:
[plain]
- //
- // HScore.h
- // dafeiji
- //
- // Created by 丁小未 on 13-9-25.
- //
- //
- #ifndef __dafeiji__HScore__
- #define __dafeiji__HScore__
- #include <iostream>
- #include "cocos2d.h"
- using namespace cocos2d;
- class HScore : public CCLayer
- {
- public:
- virtual bool init();
- static CCScene * scene();
- CREATE_FUNC(HScore);
- private:
- void backMenu();
- };
- #endif /* defined(__dafeiji__HScore__) */
HScore.cpp:
[plain]
- //
- // HScore.cpp
- // dafeiji
- //
- // Created by 丁小未 on 13-9-25.
- //
- //
- #include "HScore.h"
- #include "HMenu.h"
- using namespace std;
- CCScene * HScore::scene()
- {
- CCScene * scene = CCScene::create();
- CCLayer * layer = HScore::create();
- scene->addChild(layer);
- return scene;
- }
- bool HScore::init()
- {
- if (!CCLayer::init()) {
- return false;
- }
- CCSize size = CCDirector::sharedDirector()->getWinSize();
- //创建背景
- CCSprite * sp = CCSprite::create("score_bg.png");
- sp->setPosition(CCPointMake(size.width/2, size.height/2));
- addChild(sp);
- string scoreStr = "";
- string score = CCUserDefault::sharedUserDefault()->getStringForKey("user_score","-1").c_str();//如果没有值的话就默认为-1
- //atoi接受的是一个c风格的字符串返回的是一个整形
- //c_str是返回一个C类型的字符串
- if (atoi(score.c_str())!=-1) {
- scoreStr+=score;
- }
- else{
- scoreStr = "0";
- }
- //分数
- CCLabelTTF * ttfAbout = CCLabelTTF::create(scoreStr.c_str(), "Helvetica", 23);
- ttfAbout->setPosition(CCPointMake(size.width * 0.5 - 50, size.height * 0.5 + 40));
- ttfAbout->setColor(ccRED);
- this->addChild(ttfAbout);
- //返回主菜单
- CCLabelTTF * ttback = CCLabelTTF::create("返回主菜单","Helvetica", 23);
- //设置为黄色
- ttback->setColor(ccc3(255, 255, 0));
- CCMenuItemLabel *menuLabel = CCMenuItemLabel::create(ttback, this, menu_selector(HScore::backMenu));
- menuLabel->setPosition(CCPointMake(0, -200));
- CCMenu * menu = CCMenu::create(menuLabel,NULL);
- addChild(menu);
- return true;
- }
- void HScore::backMenu()
- {
- //自上而下翻滚切换
- CCDirector::sharedDirector()->replaceScene(CCTransitionFadeDown::create(1.5, HMenu::scene()));
- }
8.关于作者场景的设计:
HAbout.c:
[plain]
- //
- // HAbout.h
- // dafeiji
- //
- // Created by 丁小未 on 13-9-25.
- //
- //
- #ifndef __dafeiji__HAbout__
- #define __dafeiji__HAbout__
- #include <iostream>
- #include "cocos2d.h"
- using namespace cocos2d;
- class HAbout : public CCLayer
- {
- public:
- virtual bool init();
- static CCScene * scene();
- CREATE_FUNC(HAbout);
- private:
- void backMenu();
- };
- #endif /* defined(__dafeiji__HAbout__) */
HAbout.cpp:
[plain]
- //
- // HAbout.cpp
- // dafeiji
- //
- // Created by 丁小未 on 13-9-25.
- //
- //
- #include "HAbout.h"
- #include "HMenu.h"
- #include "SimpleAudioEngine.h"
- using namespace CocosDenshion;
- CCScene * HAbout::scene()
- {
- CCScene * scene = CCScene::create();
- CCLayer * layer = HAbout::create();
- scene->addChild(layer);
- return scene;
- }
- bool HAbout::init()
- {
- if (!CCLayer::init()) {
- return false;
- }
- CCSize size = CCDirector::sharedDirector()->getWinSize();
- //添加背景
- CCSprite * sp = CCSprite::create("score_bg.png");
- sp->setPosition(CCPointMake(size.width/2, size.height/2));
- addChild(sp);
- //添加返回菜单
- CCLabelTTF * ttback = CCLabelTTF::create("返回主菜单", "Helvetica-bold", 23);
- ttback->setColor(ccc3(255, 255, 0));
- //返回菜单项
- CCMenuItemLabel * menuLabel = CCMenuItemLabel::create(ttback, this, menu_selector(HAbout::backMenu));
- menuLabel->setPosition(CCPointMake(0, -200));
- CCMenu *menu = CCMenu::create(menuLabel,NULL);
- addChild(menu);
- return true;
- }
- //返回主菜单
- void HAbout::backMenu()
- {
- //翻页切换
- CCDirector::sharedDirector()->replaceScene(CCTransitionPageTurn::create(1.5, HMenu::scene(), true));
- }
运行效果:


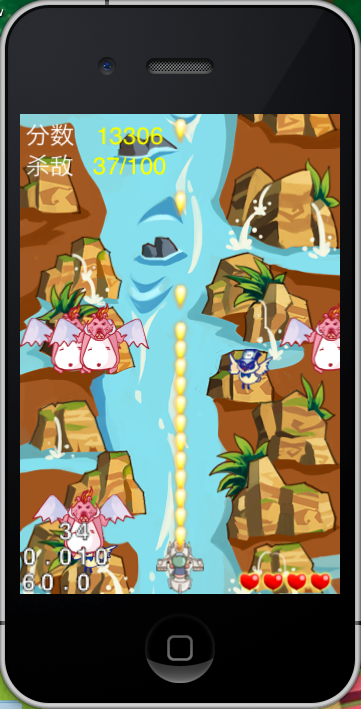
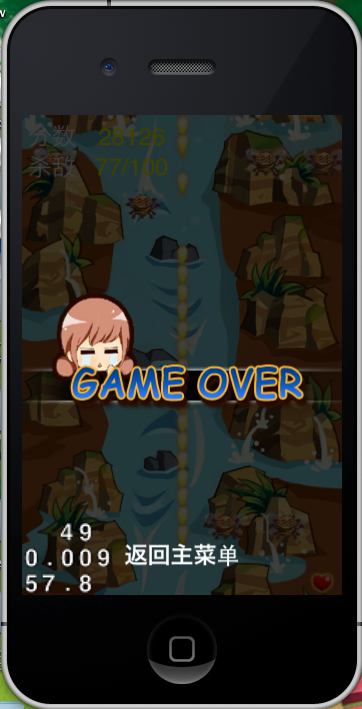
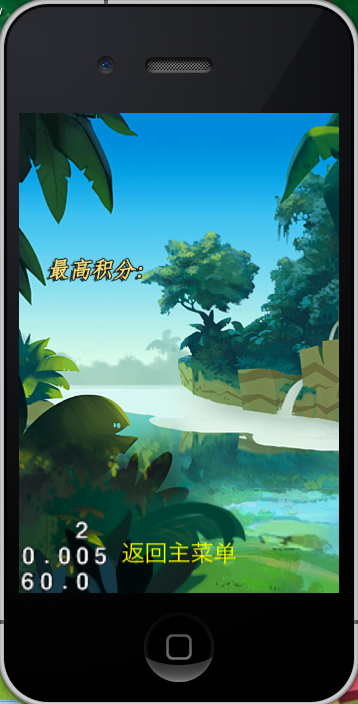
如何修适应安卓机?
1.在AppDelegate的applicationDidFinishLaunching方法中添加这么一行屏幕分辨率自适应:
CCEGLView::sharedOpenGLView()->setDesignResolutionSize(320,480,kResolutionUnKnown);
2.还有在eclipse中修改一个AndroidManifest.xml文件,
屏幕默认是横屏的,如果要修改成纵屏,则修改android:screenOrientation="portrait"
项目源码:http://download.csdn.net/detail/s10141303/6334027
已经编译成的安卓项目:http://download.csdn.net/detail/s10141303/6422971
或者加群获得源码
cocos2d-x游戏开发QQ交流群:280818155
备注:加入者必须修改:地区-昵称,很少有动态的将定期清理